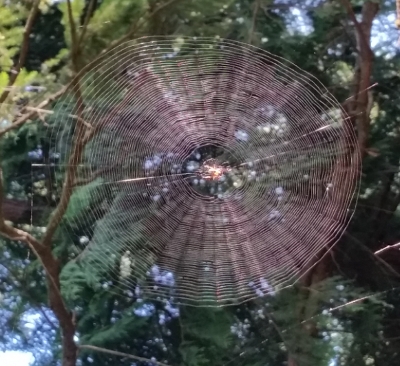
Iteration
Example 1 - for
C
#include
<stdio.h>
int main () {
for(int
x=1;x<10;x++){
puts("hello");
}
}
PHP
<?php
for ($x=1; $x<10; $x++) {
echo "hello";
}
?>
Python
x = 1
while x < 10:
print("hello")
x += 1
for _ in range(1,10):
print("hello:",_)
Bash
#!/bin/bash
for (( x=1; x<=10; x++ ))
do
echo "Welcome $x times"
done
Example 2 - while
C
#include
<stdio.h>
int main () {
int x = 1;
while (x++ < 10) {
puts("hello");
}
}
or
#include
<stdio.h>
int main () {
register int x = 1; // Look at the difference in the .asm
code
// gcc -o while.asm -S while1.c
while (x++ < 10) {
puts("hello");
}
}
PHP
<?php
$x = 1;
while ($x < 10){
print("hello");
$x += 1;
}
?>
Python
x = 1
while x < 10:
print("hello")
x += 1
Bash
#!/bin/bash
x=0
while [ $x -lt 10 ]
do
echo hello
x=$[$x+1]
done
Note: I have not done any input in the above examples.
Input of course can be done the same way as we did for Selection.
Hands on
- The same as the previous hands on. Copy the 4 files into
iteration1.c, etc. and run them.
- ssThen do it again for the second set of examples using
iteration2.c etc.
Then:
Modify the one of the programs to take an input of the number of
times to loop.
Home Work
Modify (or create new) all 8 programs to take input
Choose one of the languages and write a program to display the
first n fibonacci numbers. Where in is input.
A fibonacci number is the sum of the previous 2 numbers
- The first number is 0
- The second number is 1
- The third number is 1
- The forth number is 2
- The fifth number is 3
- etc...