Javascript and html5
All of the following examples
were tested using a Galaxy S20 FE 5G using the Chrome web
browser. Your results may vary.
I will be covering:
- Get GPS co-ordinates -
- Phone Orientation
- Phone Acceleration
- Canvas and plotting
There are examples and code for each of the above.
Note: that for the GPS and phone sensor data the
JavaScript must be served from an https page.
Note: I have done little to no defensive programming with
this code.
If you want to let this loose on the world, you should do that:
- Does the device have the sensor you are trying to use?
- Do you have permission to use it?
- Is it working as you expect?
- Does it support canvas?
- Does it support GPS
... Things like that.
Links: http://deid.ca/ps/links.html
Links to all the URLs mentioned in the presentation to save typing
them into your phone
Calculate and Display Gas Mileage
I created this system in 2014 when we bought our Prius C. I
wanted to check if the mileage calculations the Prius was doing
were accurate. It turns out they were so I kind of lost
interest in it. I became interested again when I was
thinking of things I could measure with my phone.
Examples - note that all data is made
up
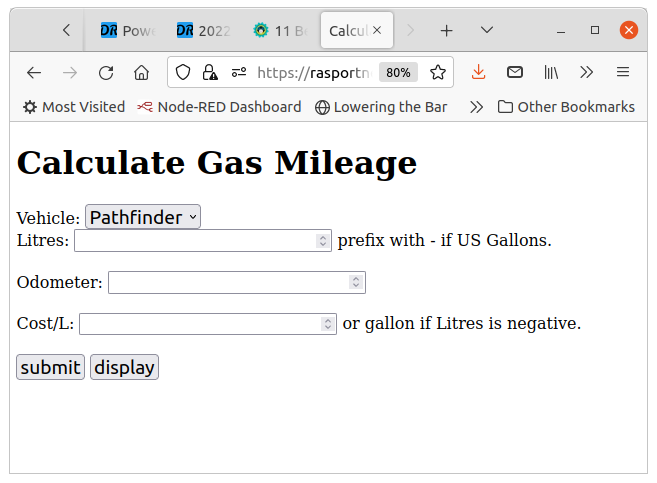
Data Entry
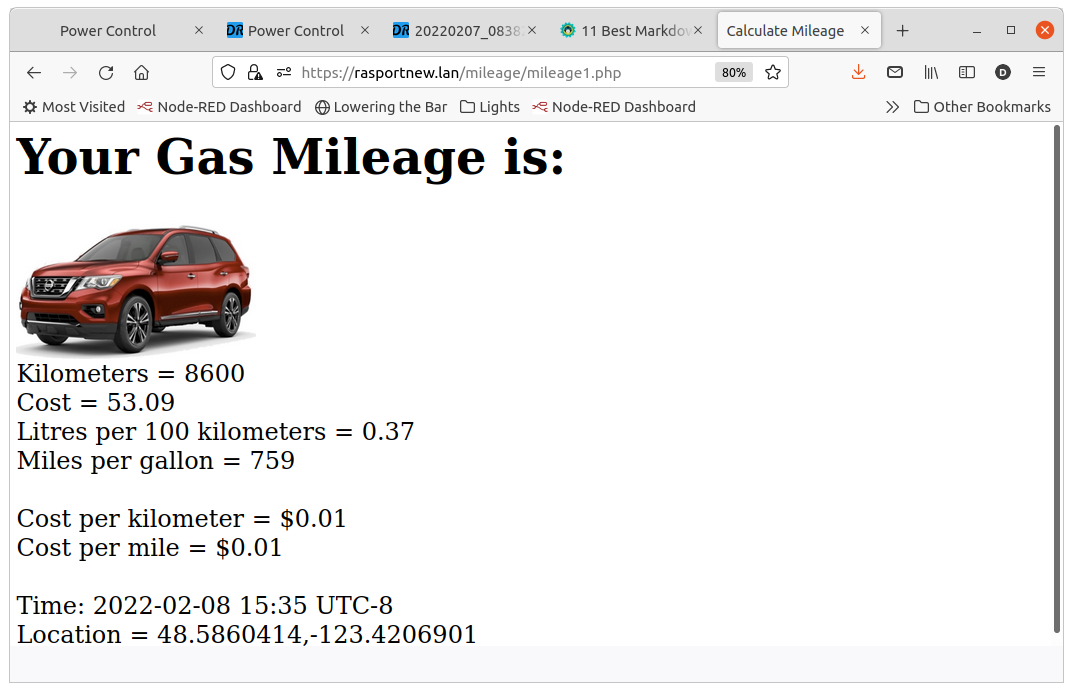
Calculation for Pathfinder
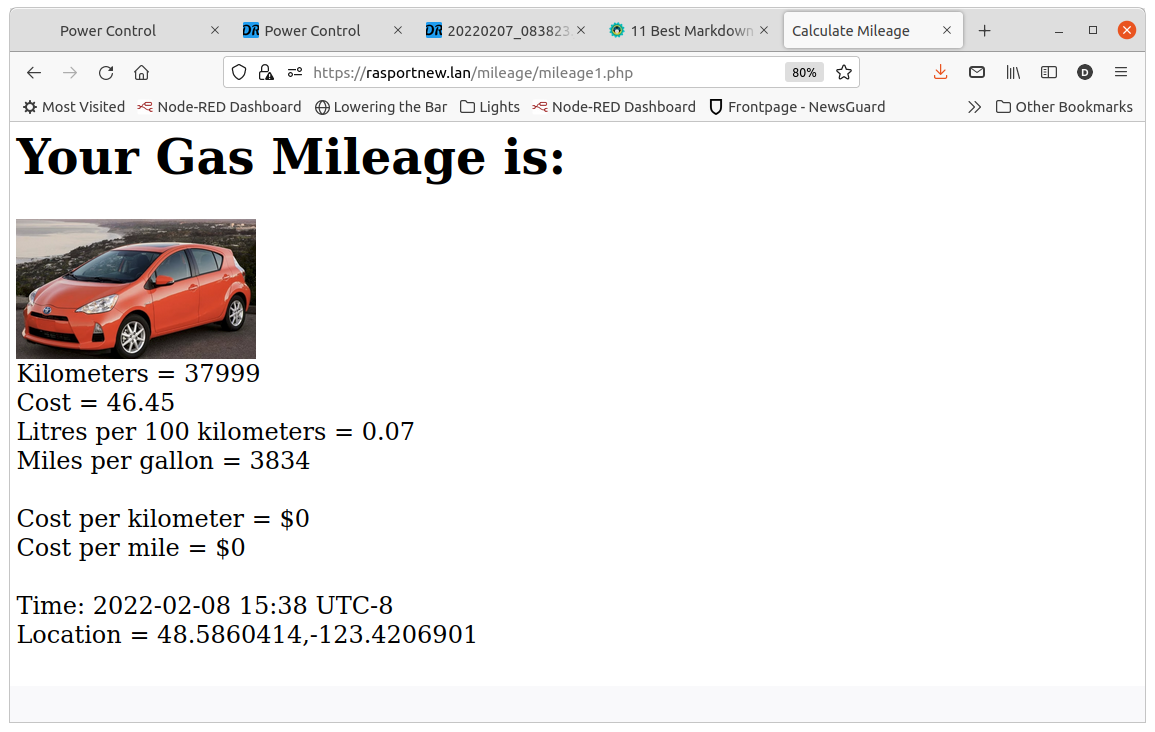
Calculation for Prius
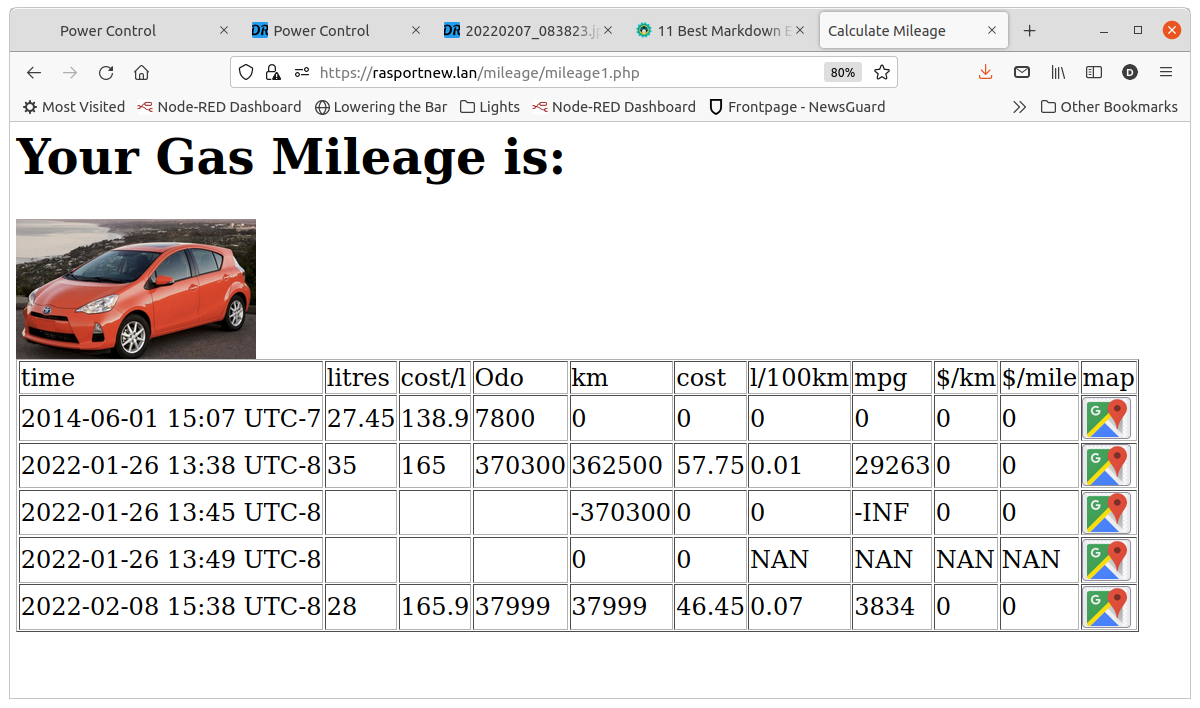
Display of all entries for the Prius
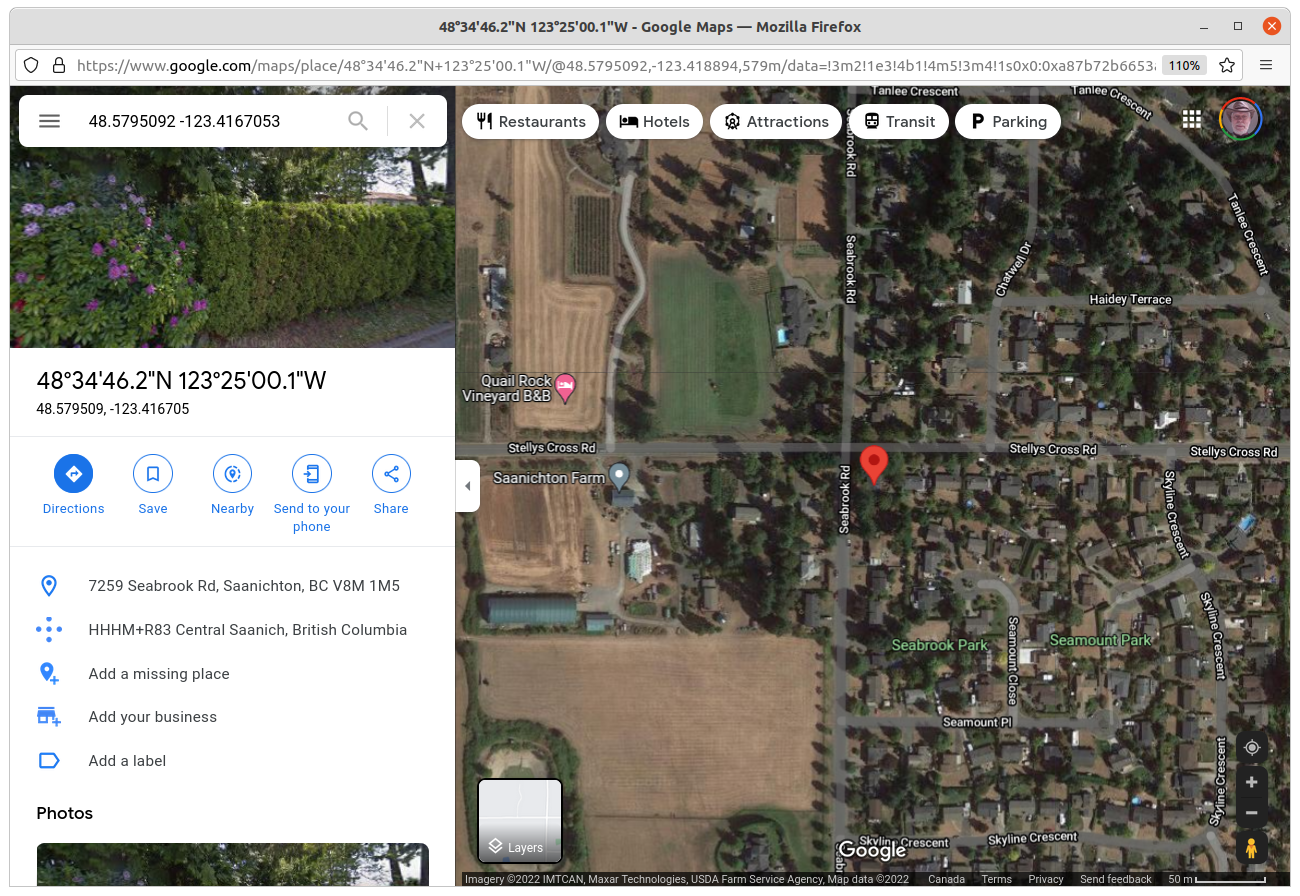
Display of the Link to Google Location Note this is from an
entry from a device that actually has a GPS. Entering the data
from my desktop creates imaginative results.
The intent is to enter the data as I am getting gas so that the
google image shows where I was when I got gas.
Source Code
HTML Code: mileage1.html
PHP Code: mileage1.php
How to do this - on a Pi - Summary
Details at https.html
-
Install a web server, php and ssl on my PI
-
Test that it can serve a web page
-
Configure apache
-
Install the code
Device Orientation and Motion Sensor
I created three simple web pages to test these sensors
Orientation
Because of my flying background I like to call this:
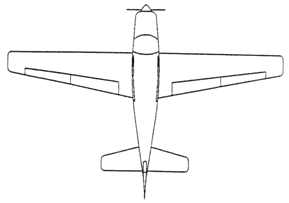
- Yaw (α) Alpha
- Pitch (β) Beta
- Roll (γ) Gamma
Orientation measures and this code displays the orientation of
the phone in space showing the Yaw Pitch and Roll in degrees.
URL: https://deid.ca/ps/pi/motion/orientation.html
Documentation
https://developer.mozilla.org/en-US/docs/Web/API/Window/deviceorientation_event
Code: orientation
Acceleration
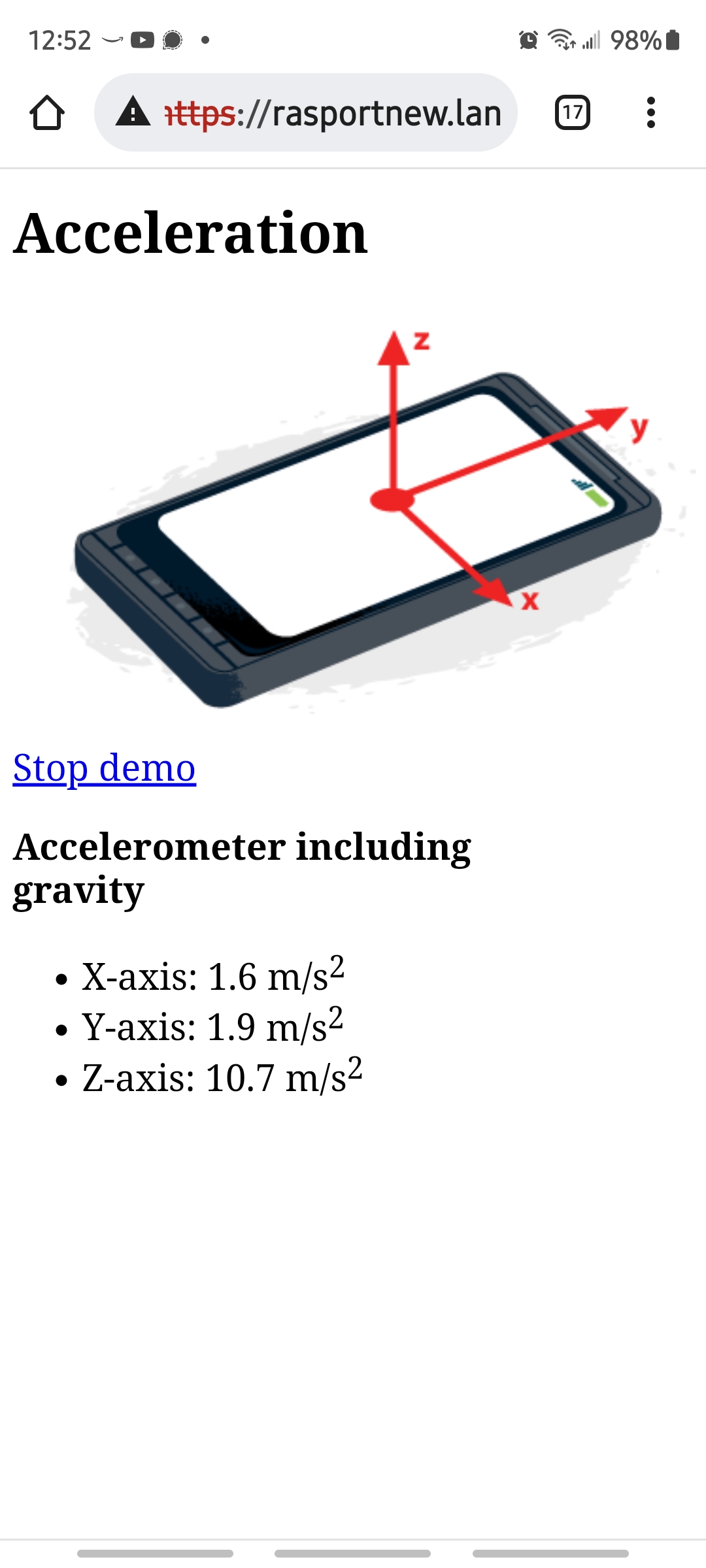
Acceleration measures the acceleration in metres/sec. for the X,
Y and Z axis. There are two ways of doing this - including
gravity and excluding gravity. This example shows the
acceleration with gravity.
URL: https://deid.ca/ps/pi/motion/acceleration.html
Documentation
Code: acceleration
Max Acceleration
This code simply calculates and displays the maximum acceleration
since start. It is kind of hard to see what is happening in
the previous acceleration so I added this.
A game: how fast can you accelerate your phone
without hurling it through a window?
URL: https://deid.ca/ps/pi/motion/accelerationmax.html
Documentation: See acceleration above
Code: accelerationmax
Canvas
Drawing with JavaScript.
My intention in delving into this was to draw a plot of the X, Y, Z
acceleration measured by the phone. And surprisingly, after a
lot of Googling and experimenting, I succeeded. I broke the
experiments into 4 parts:
- Draw a simple graph.
Code: squared
URL: https://deid.ca/ps/pi/canvas/squared.html
- Draw something moving.
Code: Ball
URL: https://deid.ca/ps/pi/canvas/ball.html
(Thanks Mozilla)
- Draw a more complex graph of a bunch of random numbers.
Code: Random
URL: https://deid.ca/ps/pi/canvas/random.html
- Draw a moving graph gathering and displaying the acceleration
on the 3 axis of my phone.
I include a picture for this as well as the link. It won't
work on a desktop as there are no sensors.
Code: Motion
URL: https://deid.ca/ps/pi/canvas/motion.html
References
2022-03-02